How it works
Python is a high-level programming language that is versatile for everything from website development to operating system applications.
The Python programming language uses interchangeable code modules instead of a long list of instructions standard for functional programming languages.

It has the following features:
- It is simple and fast: Python programs are created quickly and can have 3 to 5 lines of code less than their equivalent in Java.
- It is free: It is an open-source language, so no special license is required to use it.
- A vast community supports it: Programmers continuously develop new libraries and applications, solving possible doubts in many forums.
- It is a multi-paradigm language: It combines properties of different programming paradigms, making it very flexible and easy to learn.
- Being multi-paradigm allows it to be used in different fields such as web application design or artificial intelligence.
- Multiplatform: Python is suitable for all platforms and adapts to different software (Windows or Linux, for example).
This programming language allows us to create a multitude of communication actions in a simple way, such as bots on our brand's website, automatic messages for our marketing campaigns, control GPIO inputs and outputs on an Orange Pi or Raspberry Pi, interact with the WhatsApp server with the Yowsup-cli library, and even make calls.
How to do it step-by-step
First of all, if you don't have it yet, we recommend you create an Instasent account to enjoy all its products and have an SDK for the most common programming languages.
1 - The first thing you have to do is to install the Instasent Software Development Kit (SDK).
2 - The next step is to import the library:
3 - Then, it is time to create the client to which we want to send the SMS. In this code, you have to make sure to include your API token.
4 - Then we have to send the SMS. You can do it with Unicode characters (with which you can send emojis) or GSM (limited to Latin characters, including the ñ). Let's see an example of each one:
Unicode:
GSM:
5 - Lastly, we'll only have to check the response:
Example
This is the simplest example of sending an SMS via cURL. Here, in the "Authorization" section, you will have to put your API token:
The response:
Considerations and Recommendations
Sender
The "from" field is where the message's sender will appear to the recipient. Remember that this field has an 11 characters limit, so if the name of your brand is more prolonged, we recommend that you "trim" it, for example, by joining the words together.
Recipient
When you insert the number of one of your contacts, remember that it must always be in E.164 format. As you know, each country has a prefix that we must include when we make a call or send a message. Spain's prefix is 34. Therefore, follow these steps:
- insert the plus sign (+)
- then add the prefix of the country to which your SMS is addressed
- lastly, enter the phone number
Character set
As mentioned above, the encoding types differ depending on what you want to use in your SMS:
- GSM: This sticks to the Latin alphabet only. It includes some accents, but not all.
- Unicode: There is no limitation whatsoever, and, in addition, you can insert emoticons 😀.
When you write your SMS, Instasent will pick up the type of symbols you are using.
Message length
Depending on the character set you use, you will have a different margin in terms of the length of your text message. Think carefully before using one of the two because the difference between them is crucial:
- GSM: The maximum number of characters is 160 (153 for concatenated SMS).
- Unicode: This is limited to 70 characters (67 for concatenated SMS).
If you exceed the number of characters, the system will detect it as several SMS (regardless of the character set you use). The recipient will receive it as a single SMS (all together), but you will be counted (and charged) as several. Keep this in mind to avoid surprises.
Postman collection
In the Instasent Documentation section, you will have at your disposal the InstasentAPI.postman_collection.json folder so you can include it in Postman to use the API.
Python SDK
Instasent provides SKD to encapsulate and facilitate the use of our Rest API in several languages, including Python.
The first thing to do is to install the SDK using pip:
Once we have installed the SDK, we can use it:
Be sure to include your API token in the parentheses.
Send SMS with GSM characters only:
Sending SMS with Unicode characters:
Most common technical errors
The most common errors are response codes, which will come to you as HTTP codes from the API. Let's see what each one means:
Code 201 is a positive code, meaning the message has been sent correctly.
The problem comes when the code we receive is different from that one. In this case, each code has its explanation:
- 400 Wrong Request: The body of the request has an incorrect format, or something went wrong with your request.
- 401 Authentication Failed: The token used is invalid.
- 402 No Funds: You have run out of credit in your account.
- 413 Request entity too large: Your bulk SMS has too many elements.
- 422 Validation Errors: Validation error (e.g., when the destination number is not valid).
- 429 Rate limit reached: There are too many SMS requests per minute.
One of the most common errors is 422, which tells us precisely what failed in sending the SMS. In the example below, we see that the error is that the number is invalid.
Another common error is 429, which means that we want to send too many SMS per minute. The solution to this problem would be simply slowing down the speed at which we send messages per minute.
Why do it with Instasent API?
In Instasent, we have a very friendly interface that will facilitate and speed up any programming and shipping task. Also, our HTTP API will allow you to:
- Send SMS
- Search numbers
- Verify phone numbers
For each action mentioned above, we have a test section where you can try how they work to see if they fit your needs. In addition, we also have a tool that can generate codes automatically.
Prices
We make it easy for you to pay only for the SMS you send, with rates adapted to what you really need, without registration or monthly fees. In addition, we offer you a price calculator so you can get an idea of how much it will cost to send the SMS you want to send (the price varies depending on the destination country and the amount of SMS you want to send).
We have different payment methods available, including Paypal, Credit Card, and Bank Transfer, and we work in more than 200 countries.
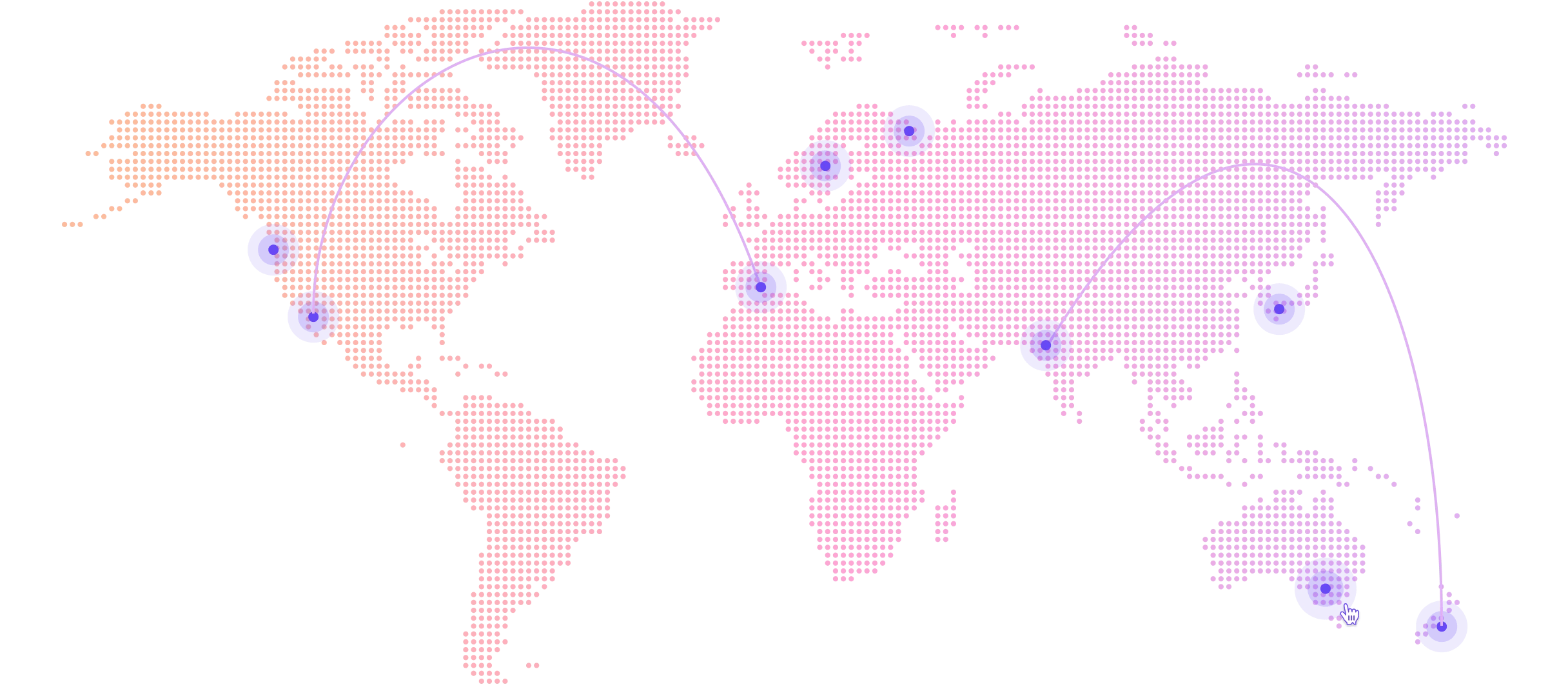