Do you want to instantly send bulk SMS to mobile phones while directly connecting to your app, website, software, CRM, etc.?
You can by using PHP.
This post will explain what PHP is and how to do it using Instasent’s API, give you tips, and show you the most common technical mistakes.
Let’s get started!
📑 In this post you will find:
What is PHP?
First of all, we need to know what PHP means.
PHP is an acronym for Hypertext Preprocessor. It is an open-source language especially suitable for web development, with which we can send mass notifications through an SMS gateway.
How does it work?
When programming with PHP, you have the opportunity to send your SMS messages from different platforms.
For example, from your website or app, or through a GSM modem, so you can send a purchase confirmation, information about errors in the service, an activation key, or an offer you have on your website.
It’s basically creating an HTML form with the following:
- A form ( form method=”post” action=”sms.php”, etc… )
- A text field to enter the sender’s name/phone number.
- A text field to enter the recipient’s phone number.
- Lastly: a field to write text (textarea) with the value “message” and a send button.
Example
Below, you can see what sending an SMS with the API would look like. Our PHP SDK does it for you using a very user-friendly interface (remember to put your API Token in the “Authorization” heading):
$> curl --location --request POST 'https://api.instasent.com/sms/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer MY_TOKEN' \
--data-raw '{ "from":"Instasent", "to":"+34666000000", "text":"Test message" }'
The response:
json
{
"entity": {
"id": "588875a72c98d52a6348e701",
"clientId": null,
"status": "enqueued",
"statusCode": null,
"from": "Instasent",
"country": "ES",
"to": "+34666000000",
"normalizedTo": "+34666000000",
"charsCount": 12,
"text": "Test message",
"deliveredText": "Test message",
"messagesCount": 1,
"concatsLimit": 10,
"finalMessagesCount": 1,
"encoding": "GSM_7BIT",
"unicode": false,
"allowUnicode": false,
"isSanitized": true,
"isTruncated": false,
"charged": true,
"pricePerSms": 0,
"priceUser": 0,
"deliveryReportMask": 0,
"scheduledAt": null,
"chargedAt": "2020-01-01T00:00:00+0200",
"sentAt": null,
"deliveredAt": null,
"createdAt": "2020-01-01T00:00:00+0200"
}
}
How to do it through Instasent’s API
Step 1: Install Instasent’s SDK
$> composer require instasent/instasent-php-lib
php
<?php
use Instasent\SmsClient as InstasentClient;
Step 2: Import library
require __DIR__ . '/vendor/autoload.php';
Step 3: Create a client
Make sure to add here your API token:
$instasentClient = new InstasentClient("MY_TOKEN");
Step 4: Send an SMS
$response = $instasentClient->sendSms("Instasent", "+34666000000", "Test message");
Alternative Step 4: Send SMS with Unicode characters (with this option, you can send emojis).
$response = $instasentClient->sendUnicodeSms("Instasent", "+34666000000", "Unicode test message: ña éáíóú 😀");
Step 5: Review the response
if ($response["response_code"] === 201) {
// Send success
$sms = \json_decode($response["response_body"], true)['entity'];
} else {
// Send error
}
Recommendations and tips when sending messages by PHP
Sender
When you want to send a text message, you must include the sender, which will be in the “from” field. The name or number you put here will be the one that appears to the recipient.
However, keep in mind that this field has a limit of 11 characters.
Recipient
The recipient’s phone number must be in the E.164 format and must include:
- The plus sign (+)
- Followed by the country prefix (for example, 34 for Spain)
- Lastly: the telephone number
Character set
It is essential to take into account the set of characters of your SMS message:
– GSM (Global System for Mobile Communications): This system is limited to Latin characters, including ñ, and only certain accents.
– Unicode: The allowed characters are unlimited, and you can even send emojis 😀.
Please note that Instasent’s platform automatically detects the character set used.
Message length
As we have already discussed in previous posts, SMS are limited in length, and this length depends on the character set used:
- GSM: Has a limit of 160 characters per message (153 for concatenated messages).
- Unicode: The limit of characters a message can fit goes down to 70 (67 for concatenated messages).
A message whose length exceeds the limit to fit in a single SMS, both for GSM and Unicode, will be split into several parts and sent to the desired phone number.
Once all the parts reach the phone, the original message is recomposed so that it finally appears as a single message to the recipient.
Postman collection
We provide the InstasentAPI collection in the InstasentAPI.postman_collection.json file, to include it in Postman so you can quickly test the API.
To help you see how our API works, we provide a Postman collection that you can download.
Response codes
The API uses HTTP codes for its responses.
Code 201 is the one that indicates that the SMS has been sent successfully.
In case you receive a code other than 201, here is a summary of the possible meaning:
- 400 Wrong Request: There is something wrong with the request.
- 401 Authentication Failed: The token used is not valid.
- 402 No Funds: No balance for sending messages.
- 413 Request entity too large: Too many elements in a bulk mailing.
- 422 Validation Errors: Validation error.
- 429 Rate limit reached: Too many requests per minute.
Most common errors
As we have just seen, there can be a number of errors when sending a text message via PHP, but there are two of them that are most common:
- 422 – the most common error, in whose response is indicated the field or fields whose validation has failed:
json
{
"errors": {
"fields": {
"to": [
"Invalid number"
]
}
}
}
- 429 (too many requests per minute), the second most common error. In this case, the solution is simply to lower the sending speed per minute.
Optimizing Your SMS Integration in PHP
Once you’ve mastered the basics of sending SMS using PHP, the next step is optimizing your sms integration in php. This involves ensuring efficient handling of SMS delivery statuses, managing error responses, and fine-tuning the system for high-volume messaging.
Effective integration can significantly enhance the functionality of your PHP application, offering a more robust and user-friendly communication platform. It’s also essential to stay updated with the latest PHP practices and SMS API updates to maintain optimal performance and security.
Why should you send your texts with Instasent’s API?
Instasent’s HTTP API allows you to send text messages through a very user-friendly interface while also being able to do number searches.
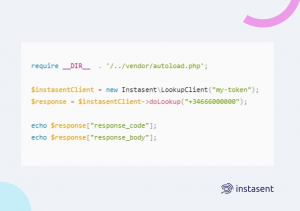
At Instasent, we even have a test section on each endpoint, so you can quickly test API requests/responses.
We also have some SDKs for the most popular programming languages, as well as an automatic code generation tool.
Our prices
At Instasent, we provide rates to suit your needs. That’s why you only pay for the SMS you send, with no joining or monthly fees.
You can check our price calculator here, which depends on the destination country and the amount of SMS you want to send.
If you want to send 300 SMS to Spain, for example, the price would be 12€, as you can see in the chart below.
PERSONALIZED QUOTE
PURCHASE SUMMARY
COUNTRY Spain
No. of SMS 0
Total Price 0 €
Price for each SMS 0 €
Download the complete list of SMS prices according to countryWOW!
For quantities of more than 10,000 SMS, get in touch with us and we’ll make you a great offer!